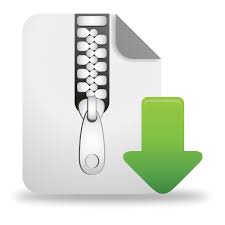
Task 1: Simple Conditionals, Task 2: More Conditionals
The first task of this lab is to write a program that check that if the given three points ( , ) can be a right triangle.
A right triangle is the one that has an angle of 90 degrees, and can be verified by the following formula:
2= 2+2
where , , and are the lengths of three sides of the triangle.
The data segment is declared as follows:
- .data
2
side_a:
.quad
3
3
side_b:
.quad
4
4
side_c:
.quad
5
5
yes:
.string
"It is a right triangle.\n"
6
len_yes:
.quad
. - yes
// Calculate the length of string yes
7
no:
.string
"It is not a right triangle.\n"
8
len_no:
.quad
. - no
// Calculate the length of string no
where you can assume side_c is always the longest side. If it is a right triangle, you’d need to print the string
"It is a right triangle.\n " ; otherwise "It is not a right triangle.\n " .
To print a string, you might want to review and borrow the code from lab 2.
Requirements
Note your code is a complete assembly program (not just a sequence of instructions). It should be able to assemble, link, and execute without error and warnings. When executed, the program should finish without problems. If your code cannot assemble, you get no credit – this is the same as C programs that cannot be compiled;
MUL instruction can be used for multiplications;
You can use either CBZ / CBNZ or B.EQ / B.NE for branching; Avoid using registers X29 and X30;
You have to put comments on each line of instruction;
Put your name and honor code pledge at the top of your code in comments.
- Task 2: More Conditionals
This task is to get familiar with other conditional branching instructions such as B.LE . You are given the starter code as follows (it can also be downloaded from Canvas):
- .text
- .global _start
3 .extern scanf
- Grading
The lab will be graded based on a total of 10 points, 5 for task 1 and 5 for task 2. The following lists deductibles, and the lowest score is 0 – no negative scores:
Task 1:
- -5: the code does not assemble, or the program terminates abnormally/unsuccessfully;
- -5: the code is generated by compiler;
- -5: no output is printed;
- -5: not using conditionals;
- -3: the right triangle checking result is wrong;
- -1: one or more instructions is missing comments;
- -1: no pledge and/or name. Task 2:
- -5: the code does not assemble, or the program terminates abnormally/unsuccessfully;
- -5: the code is generated by compiler;
- -5: no output is printed;
- -5: not using conditionals;
- -1: 1 point off for each of the following test cases:
* -563;
- -5 10 -30 ;
- -6 -2 0;
- 343;
- 10 30 30;
- -20 20 -20 ;
- -1: one or more instructions is missing comments;
- -1: no pledge and/or name.
Earlybird Extra Credit: 2% of extra credit will be given if the lab is finished by Wednesday 11:59PM EST (1 day before the lab deadline). For specific policy, see syllabus.
Attendance: check off at the end of the lab to get attendance credit.
Deliverable
Assembly code for both tasks in two separate .s files. No need to zip all files; just submit both files separately on Canvas.
3