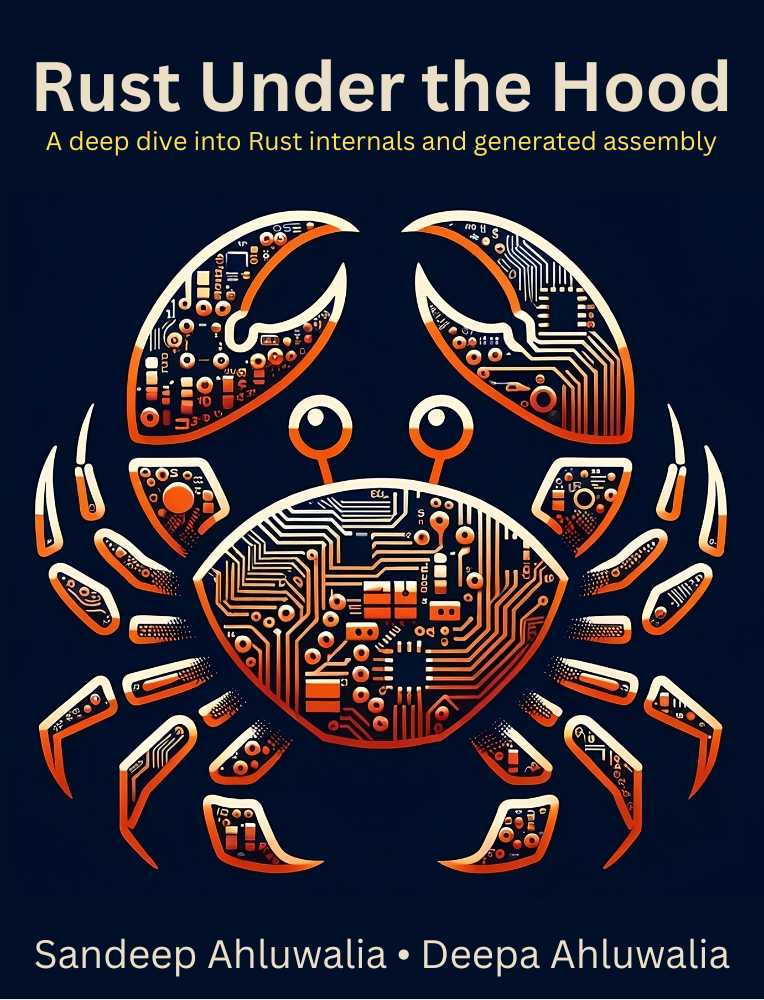
Rust Under the Hood
Rust Under the Hood
Unlock the full potential of the Rust programming language with "Rust Under the Hood."
This comprehensive guide delves into Rust's core mechanics by examining the assembly code generated by its compiler. Ideal for Rust enthusiasts of all levels, this book offers unique insights into the generated code, memory management, and compiler optimizations.
Discover How Rust Works Under the Hood
- Learn how Rust represents, and pattern matches enums, tuples, and structs.
- Dig into implicit heap operations with Box and Vec.
- Understand string and &str representation and generated code.
Understand Dispatch Mechanisms
- Compare and contrast static vs. dynamic dispatch and the pivotal role of Vtables.
- Grasp how closures interact with their environments and manage state.
Rust Compiler Optimizations and SIMD
- Understand loop optimization and SIMD auto-vectorization.
- Explore strategies used for branch avoidance and function inlining.
- Learn how tail call optimization transforms recursive functions into loops.
Async Programming Insights
- Learn how Rust transforms async functions into state machines.
- Unravel the workings of async executors and the role of polling in async/await constructs.
Practical Learning
"Rust Under the Hood" pairs insightful explanations with practical examples and exercises at the end of each chapter. Use tools like Compiler Explorer and Rust Playground to reinforce your learning and gain hands-on experience with the generated x86-64 assembly.
Who Should Read This Book?
- Beginners to Rust: Gain a foundational understanding of Rust's high-level constructs and their low-level representations. Follow detailed flowcharts and memory diagrams to grasp the flow of the generated code and understand the memory management aspects without diving deep into assembly code.
- Experienced Rust Developers: Gain insights into the generated code's nuances and memory representations through annotated assembly code, aiding you in writing more efficient Rust programs.
- C++ Developers Moving to Rust: Understand Rust's memory management, dispatch mechanisms, and compiler optimizations.
- Embedded Systems Developers: Learn how Rust generates efficient assembly code for constrained environments. Optimize your embedded applications by understanding Rust's performance and memory management strategies.
Book Structure
The book consists of seven parts, each focusing on various aspects of Rust's inner workings:
- Introduction to Assembly and Basic Constructs: Start with the basics of assembly language and see how Rust translates simple functions into assembly code.
- Control Structures and Enums in Assembly: Explore the assembly output of match and if-else expressions and understand the memory layout of enums.
- Data Structures and Memory Management: Investigate the code generation nuances of various data structures and memory management techniques in Rust.
- Iteration and Optimization: Compare traditional and functional iteration methods and learn how the Rust compiler optimizes array operations.
- Strings, Dispatch, and Recursion: Dive into the inner workings of strings, dynamic dispatch, and recursive function optimization.
- Closures and Async/Await: Understand the mechanics of closures and asynchronous functions and how Rust translates them into efficient state machines.
- Transformations and Takeaways: Summarize key insights and transformations, providing a solid foundation for future Rust projects.